The ECMAScript 2015 introduced us to the concepts of let
, const
, arrow functions along with JavaScript Object Destructuring. Object destructuring is a significant feature in JavaScript. It is extensively used in front-end framework like React as well as backend services like Node.js. In this article, we'll try to cover the basics of Object Destructuring.
What is Destructuring?
Destructuring broadly means extracting data from arrays or objects. Using destructuring, we can break a complex array or object into smaller parts. Destructuring also offers us the ability to extract multiple data at once from an object or array. It also provides the ability to set a default value of the property if it's already not set.
Destructuring with Example:
Let's understand Object Destructuring with some examples.
Suppose we have an object with the following key-value properties,
let person = {
firstName: "Captain",
lastName: "Nemo"
}
Before ES6, to get the values individually, we needed to do something like this,
const firstName = person.firstName;
const lastName = person.lastName;
console.log(`Hi ${firstName} ${lastName}! Nice to meet you. ????`);
It's a small object, but imagine we have a large object having a lot of key-value pairs, do you think extracting values in such a way is an efficient solution? Our code will become very much repetitive, and we don't want to disobey the rule of 'DON’T REPEAT YOURSELF'!
The ECMA Organisation understood the problem and introduced us to the concept of Object Destructuring in JavaScript. Using destructuring, we can easily extract the properties using the following code,
const { firstName, lastName } = person;
console.log(`Hi ${firstName} ${lastName}! Nice to meet you. ????`);
This might seem confusing at first. Until now, we used curly braces on the right side. But here, the braces are on the left. This is how the destructuring syntax looks.
Basically, it just means,
Give me a variable called firstName
and a variable called lastName
from the object called person.
Destructuring a Nested Object
Object destructuring comes handier when we are working with a nested object.
Imagine an object like the below one,
const person = {
firstName: "Captain",
lastName: "Nemo",
address: {
street: "1234 Ave",
city: "Antilaw State",
zip: 1234
}
}
To access the elements using our old school approach, the code will be too repetitive and will look very dirty.
console.log(person.firstName);
console.log(person.lastName);
console.log(person.address.street);
console.log(person.address.city);
console.log(person.address.zip);
Output:
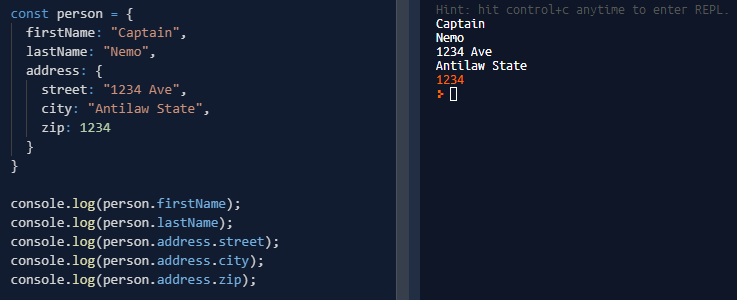
And now, let's take a look using the ES6 object destructuring,
const { firstName, lastName, address: { street, zip, city } } = person;
console.log(firstName);
console.log(lastName);
console.log(street);
console.log(city);
console.log(zip);
It is a much better approach to access the elements. And, moreover, we have to write less code.

I want to share a beautiful quote about writing shortcodes from the book Eloquent JavaScript here,
Tzu-li and Tzu-ssu were boasting about the size of their latest programs. ‘Two-hundred thousand lines,’ said Tzu-li, ‘not counting comments!’ Tzu-ssu responded, ‘Pssh, mine is almost a million lines already.’ Master Yuan-Ma said, ‘My best program has five hundred lines.’ Hearing this, Tzu-li and Tzu-ssu were enlightened.
Master Yuan-Ma, The Book of Programming
Storing Object Values to Custom Variables
Now, let's say we need to store the extracted properties to custom variables. We can also do this using object destructuring. Suppose we want to store the firstName
element of the person object to a variable called first
and the lastName
to a variable called last
. We can achieve this using the following code,
const { firstName: first, lastName: last } = person;
console.log(first);
console.log(last);
And yes, we can also extract only the elements we want. In the above code, we extracted only the firstName
and the lastName
from the object.
Destructuring Arrays
Object Destructuring can also be used to destructure arrays.
Here's an example,
Suppose an array contains scores of a student in 3 subjects.
const scores = [85, 90, 74];
We can destructure the scores easily using the following code,
const [maths, geography, biology] = scores;
console.log(maths);
console.log(geography);
console.log(biology);
So, using the simple array literal to the left, we can destructure the array. The array elements are getting stored in local variables that are defined by us. Each of the local variables will map with the corresponding array element.
Output:

Conclusion
I think this article has given you a quite understanding of object destructuring in ES6. If this article helped you or you've feedback, please comment below.
You may also like: