LAST UPDATED ON: SEPTEMBER 17, 2024
Program for Matrix Multiplication in C
Before we see the program for matrix multiplication in C, let's first know what are matrix in C and what are the different operations that we can perform on matrix in C.
What is a Matrix?
A two dimensional array is called a matrix. For example,
int arr[4][5];
float marks[5][2];
char ch[4][6];
// define just the number of columns and not rows
int arrrr[][3];
While declaring a matrix, we always define the number of columns even if we leave the number of rows empty. This is the default syntax for declaring a matrix in C. To learn more about this, check out our tutorial on Arrays in C.
Types of Matrix Operations
We can perform addition, subtraction, multiplication and division operations on matrix. Out of all those, multiplication is the most complex. Before we dive into that, let's see how we can calculate sum of individual rows of a matrix.
#include <stdio.h>
int main()
{
int arr[2][3], total[2];
for(int i = 0; i < 2; i++) {
for(int j = 0; j < 3; j++){
scanf("%d", &arr[i][j]);
}
}
for(int i = 0; i < 2; i++) {
int sum = 0;
for(int j = 0; j < 3; j++){
sum += arr[i][j];
}
total[i] = sum;
}
for(int i = 0; i < 2; i++)
printf("%d : %d\n", i + 1, total[i]);
return 0;
}
1 2 3
1 2 4
1 : 6
2 : 7
Matrix Multiplication in C Program
We may have to multiply a matrix with a number (scalar value) or we may have to multiply two matrices. So let's explore both the scenarios.
1. Multiplying Matrix with a scalar value
Matrix multiplication with a single number is easy and can be done as follows:
#include <stdio.h>
int main()
{
int arr[2][2], n;
// enter the scalar value
scanf("%d", &n);
// input the matrix values
for(int i = 0; i < 2; i++) {
for(int j = 0; j < 2; j++){
scanf("%d", &arr[i][j]);
}
}
// multiply every value of matrix with scalar value
for(int i = 0; i < 2; i++) {
for(int j = 0; j < 2; j++){
arr[i][j] = arr[i][j] * n;
}
}
// print the updated matrix values
for(int i = 0; i < 2; i++) {
for(int j = 0; j < 2; j++){
printf("%d ", arr[i][j]);
}
printf("\n");
}
return 0;
}
5
1 2 3 4
5 10
15 20
Run Code →
2. Multiplication of Two Matrices
There are two things we have to keep in mind while performing matrix multiplication on two matrices:
-
The number of columns of the first matrix should be equal to the number of rows of the second matrix.
-
The resultant matrix will have the same number of rows as the first matrix and the same number of columns as the second matrix.
Suppose we have two matrices:
A = {{1, 3, 5},
{4, 2, 6}};
B = {{7, 4},
{3, 1},
{6, 9}};
To multiply these two matrices, we will perform dot product on their rows and columns, means the first element of the firast row of A will be multiplied to the first element of first column of B, the second element of first row of A will be multiplied to the second element of first column of B, and so on.

Algorithm for Matrix Multiplication in C
The standard algorithm for matrix multiplication is as follows:
Let A be a m * k matrix, B be a k * n matrix. We will store our result in C[m][n].
for i = 1 to m
for j = i to n
c[i][j] = 0
for p = 1 to k
C[i][j] = C[i][j] + A[i][p] * B[p][j]
Below is a program on Matrix Multiplication:
#include<stdio.h>
int main()
{
printf("\n\n\t\tStudytonight - Best place to learn\n\n\n");
int n, m, c, d, p, q, k, first[10][10], second[10][10], pro[10][10],sum = 0;
printf("\nEnter the number of rows and columns of the first matrix: \n\n");
scanf("%d%d", &m, &n);
printf("\nEnter the %d elements of the first matrix: \n\n", m*n);
for(c = 0; c < m; c++) // to iterate the rows
for(d = 0; d < n; d++) // to iterate the columns
scanf("%d", &first[c][d]);
printf("\nEnter the number of rows and columns of the first matrix: \n\n");
scanf("%d%d", &p, &q);
if(n != p)
printf("Matrices with the given order cannot be multiplied with each other.\n\n");
else // matrices can be multiplied
{
printf("\nEnter the %d elements of the second matrix: \n\n",m*n);
for(c = 0; c < p; c++) // to iterate the rows
for(d = 0; d < q; d++) // to iterate the columns
scanf("%d", &second[c][d]);
// printing the first matrix
printf("\n\nThe first matrix is: \n\n");
for(c = 0; c < m; c++) // to iterate the rows
{
for(d = 0; d < n; d++) // to iterate the columns
{
printf("%d\t", first[c][d]);
}
printf("\n");
}
// printing the second matrix
printf("\n\nThe second matrix is: \n\n");
for(c = 0; c < p; c++) // to iterate the rows
{
for(d = 0; d < q; d++) // to iterate the columns
{
printf("%d\t", second[c][d]);
}
printf("\n");
}
for(c = 0; c < m; c++) // to iterate the rows
{
for(d = 0; d < q; d++) // to iterate the columns
{
for(k = 0; k < p; k++)
{
sum = sum + first[c][k]*second[k][d];
}
pro[c][d] = sum; // resultant element of pro after multiplication
sum = 0; // to find the next element from scratch
}
}
// printing the elements of the product matrix
printf("\n\nThe multiplication of the two entered matrices is: \n\n");
for(c = 0; c < m; c++) // to iterate the rows
{
for(d = 0; d < q; d++) // to iterate the columns
{
printf("%d\t", pro[c][d]);
}
printf("\n"); // to take the control to the next row
}
}
printf("\n\n\t\t\tCoding is Fun !\n\n\n");
return 0;
}
Program Output:
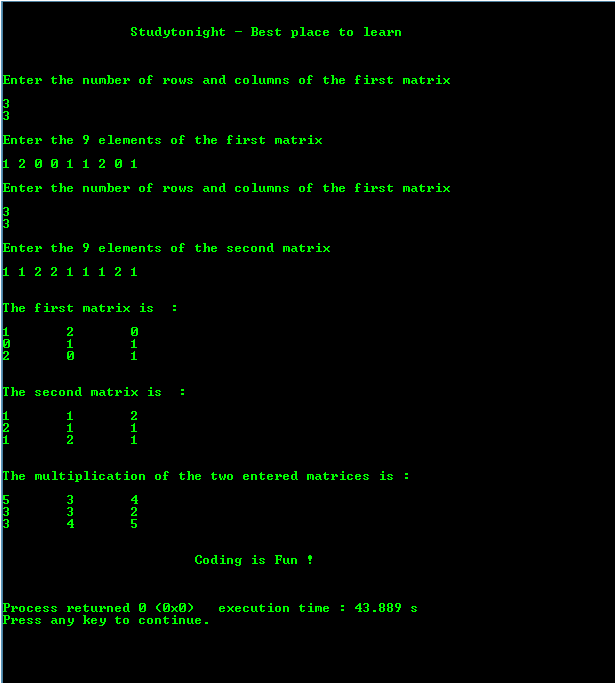