LAST UPDATED ON: SEPTEMBER 17, 2024
Hello World Program in C Language
The Hello World Program in C is the first program, just like in any other programming language, which is created to check if the basic setup and installation completed successfully or not. It is one of the simplest program you will learn.
Below is a simple program printing Hello World in C language.
//this is a comment
#include <stdio.h> //including header file in our program
int main() //main() where the execution begins
{
printf("Hello World");
return 0;
}
Hello World
Run Code →
Write the above code in a file and save it as helloWorld.c. Here .c is the file extension for C program files.
Compiling the C Program
To execute our program locally on your laptop/computer, you need to compile it first. The computer does not understand the language in which we write our code(which is close to English). In order for it to understand our code, we compile our progrm. The compiler translates our code into binary language(0s and 1s), which can then be easily understood and execited by the computer.
So, firstly, we will install a compiler in our system, let's say, gcc. To install it in your linux machine, open the terminal and type,
sudo apt-get update
sudo apt-get install gcc # install gcc
sudo apt-get install build-essential # install other required libraries
To check whether gcc
is installed successfully, check the version using the command below.
gcc --version
Now to compile our code, first go to the directory where your program is saved using the cd command.
Enter one of the following to compile your file.
gcc helloWorld.c
gcc helloWorld.c -o helloWorld # use this to name your executable file as helloWorld
All that's left is running your program like below.
./a.out # if you have compiled using gcc helloWorld.c
helloWorld # if you named your executable file as helloWorld
The output will be printed on your screen.
How does a C Program executes?
Let's see line-by-line what our C program does.
- The first line is a single-line comment. Whatever we write after // is ignored by the compiler. It is written for programmer's and user's better understanding of the code. It is not printed or used anywhere during the code's execution.
- The second line is written to include the header file stdio.h in our code. # is called the preprocessor directive. #include tells the compiler to include the header file written inside angle brackets or double quotes. The header files contain the set of predefined standard library functions to be used in our program.
- The
main()
is a function in C. Execution of a C program always begins from main()
. It is mandatory in every C program.
- The
{
is where main()
begins.
- The
printf()
is a built-in function defined in C under stdio.h header file which is used to print output on the console. It prints Hello World on the screen. It ends with a semicolon. All statements in C end with a semicolon. Otherwise, it is not considered ended. To learn more about Input/Output in C, check out our Input and Output in C tutorial.
- The
main()
is of return type int
. This means that it will return an integer and indicate finishing of the function. You can follow our tutorials to learn more about functions in C. So here, we return 0 to indicate that main()
has ended.
- } denotes end of the function. Anything between { and } is called a function's body.
We can see that our program above has been formatted, i.e., some things have been moved to the right side and nwe lines. This is called indentation. It makes understanding our code easy.
Let's see one more example where we will print some more text on the console.
#include <stdio.h>
int main()
{
printf("\n\n\t\tStudytonight - Best place to learn\n\n\n");
int num;
printf("\nHello world!\nWelcome to Studytonight: Best place to learn\n");
printf("\n\n\t\t\tCoding is Fun !\n\n\n");
return 0;
}
Output of Program:
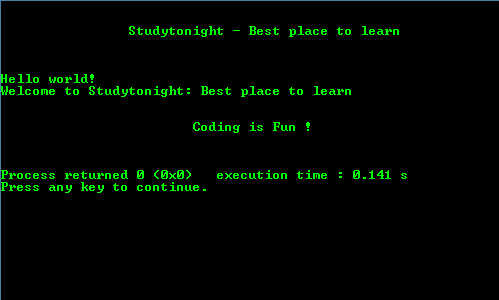
\n
is used to move the control onto the next line.
\t
is used to give a horizontal tab i.e. continuous five spaces.
Conclusion
We have written our first C program in this tutorial. To learn more, follow our complete C tutorial.