Javascript BigInt
As we know, JavaScript has only one data type for numbers which is a Number type. This data type represents a 64-bit floating-point number. It also represents the integers smaller than 2^53. To represent the numbers larger than 2^53-1, BigInt was introduced.
BigInt is a built-in object representing the whole number larger than 2^53-1, which is the largest value that JavaScript can represent. There is no limit specified as to how long a BigInt number can be and in behaviour, BigInt values are pretty similar to number values, but there is a slight difference between them. We can not use a BigInt value with a built-in Math object.
How to create BigInt?
Well, we can use the BigInt()
method to create a BigInt type object, The syntax of BigInt()
is given below:
BigInt()
Example:
<!DOCTYPE html>
<html>
<head>
<title>BigInt</title>
</head>
<body>
<script>
const bigNum = BigInt(9007199254740991)
console.log(bigNum);
const hugeStr = BigInt("9007199254740991")
console.log(hugeStr);
const hexNum = BigInt("0x1fffffffffffff")
console.log(hugehex);
const octalNum = BigInt("0o377777777777777777")
console.log(octalNum);
const binaryNum = BigInt("0b11111111111111111111111111111111111111111111111111111")
console.log(binaryNum);
</script>
</body>
</html>
Output:
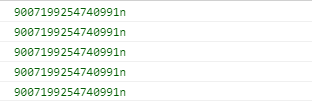
Using Operators with BigInt
BigInt type supports all JS operators especially these +
, *
, -
, %
, **
. BigInt also supports the bitwise operator except for the >>>
and unary +
operator. The divide operator (/
) will also work with the whole number,s but the result will not be any fractional digit.
Example:
In this example, we have divided the two whole numbers using the division operator (/
) which returns the rounded value 2 instead of 2.5. This is because all the operations performed on BigInt return BigInt.
<!DOCTYPE html>
<html>
<head>
<title>BigInt</title>
</head>
<body>
<script>
const wholeNum = 5n / 2n
console.log(wholeNum);
</script>
</body>
</html>
Output:

Comparison of BigInt
A BigInt is not strictly equal to a number, but it can be loosely equal. This means when we compare two BigInt values using strictly equal to (===
), it results in false, but when we compare the same values using loosely equal to (==
), it will display the result to true.
Let's understand it better using examples.
Example:
<!DOCTYPE html>
<html>
<head>
<title>BigInt</title>
</head>
<body>
<script>
console.log(2n === 2);
console.log(2n == 2);
</script>
</body>
</html>
Output:
As we can see, the output of the first expression is false, and the second expression is true.

Conditionals in BigInt
A BigInt value behaves like a number value in the following cases:
-
When the BigInt value is converted to a boolean value using the boolean()
function
-
When used with the logical operators such as ||
, &&
, and !
.
-
Within a conditional test such as if()
statement.
Example
<!DOCTYPE html>
<html>
<head>
<title>BigInt</title>
</head>
<body>
<script>
let count = 0n;
if(count) {
console.log("Hello");
}
else {
console.log("Bye");
}
</script>
</body>
</html>
Output:
In the example above, the BigInt value acts as a number and its value is treated as 0, hence, the else block is executed, because 0 value translates to false in if-else statement.

BigInt with typeof
operator
The type of a BigInt is BigInt. So when we use the typeof
operator with the BigInt to know the data type of BigInt, it will return the BigInt type.
Example:
Here is a simple code example,
<!DOCTYPE html>
<html>
<head>
<title>BigInt</title>
</head>
<body>
<script>
console.log(typeof BigInt(100) === 'bigint');
console.log(typeof 1n === 'bigint')
</script>
</body>
</html>
Output:

Conclusion
The BigInt()
method was introduced in ECMAScript 2020 or ES11. This method is used to represent the whole numbers bigger than 2^53 - 1. This method is used to create values which are used to perform complex mathematical calculations.