Javascript Promise.allSettled() method
The Promise is an implementation of asynchronous operations, and it is used to manage asynchronous operations. In JavaScript, each Promise consists there states:
-
Fulfilled or resolve (for successful operations)
-
Rejected (for failure operation)
-
Neither fulfilled nor rejected
Before moving on, you should introduce yourself to Javascript Promise and Methods to handle the states of Promise.
The methods implemented with the Promise class in ES6 or ES2015 are all()
, reject
, resolve
, etc. While the allSettled()
method is introduced in ECMAScript 2020.
The Promise.allSettled()
method accepts a list of promises and returns a new promise which resolves after all the input promises have settled, either resolved or rejected.
In simple words, we can say that this function can be used to monitor and report the status of a list of promises.
This method is used when we have multiple asynchronous tasks. These asynchronous tasks are not dependent on each other to complete successfully. This method can also be used when we want to know the result of each Promise.
In comparison, the Promise returned by Promise.all()
is used when the tasks are dependent on each other.
Syntax for allSettled()
:
Here is how we can use this function,
Promise.allSettled(iterable);
Parameter:
- An iterable is an object such as an array in which each member is a promise.
Return Value:
When we pass an empty iterable as an argument, Promise.allSettled()
method returns a Promise object which has already been resolved as an empty array.
For each returned object, there is a status string. If the value of the status attribute is fulfilled, then the value attribute is present, and if the value of the status attribute is rejected, then there is a reason attribute.
The value (or reason) attribute determines whether each Promise was fulfilled (or rejected).
Example:
In the given example we have used the Promise.allSettled()
method to wait for all the input promises to settle.
<!DOCTYPE html>
<html>
<head>
<title>Promise.allSettled() method</title>
</head>
<body>
<script type="text/javascript">
const p1 = new Promise((resolve, reject) => {
setTimeout(() => {
console.log('The first promise has resolved');
resolve(10);
}, 1 * 1000);
});
const p2 = new Promise((resolve, reject) => {
setTimeout(() => {
console.log('The second promise has rejected');
reject(20);
}, 2 * 1000);
});
Promise.allSettled([p1, p2])
.then((result) => {
console.log(result);
});
</script>
</body>
</html>
Output:
As we can see in the output image, the first Promise p1 fulfilled the value 10 after 1 second, and the promise p2 was rejected to a reason 20 after 2 seconds.
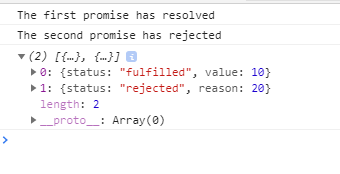
Conclusion:
We have learned about the Promise.allSettled()
method introduced in ECMAScript 2020. This method accepts a list of promises and returns a new promise, that resolves once every input Promise has settled, either rejected or fulfilled.