Javascript Nullish Coalescing Operator
Nullish coalescing was introduced in ECMAScript 2020. It is denoted by ??
double question marks.
This operator is an improved version of the logical OR operator (||
). The nullish coalescing operator works very much like the logical OR operator. The only difference is that it checks whether the value is nullish instead of falsy. This means that the operator will return its right-hand side operand if the left-hand side values are null and undefined.
Here is a table listing what all are considered as Falsy values and what all are nullish values.
Falsy Values |
Nullish Values |
Undefined |
Undefined |
Null |
Null |
0 |
- |
False |
- |
NaN |
- |
If used in if...else statement, the falsy value is treated as false.
The nullish coalescing operator has the fifth lower operator precedence, which is directly lower than the logical OR operator and higher than the conditional operator.
Syntax for Nullish Coalescing:
Here is the syntax for using nullish coalescing operator:
left_expression ?? right_expression
Let's understand it with the help of an example
Using Nullish Coalescing operator:
As mentioned above, the nullish coalescing operator sets the fallback values only for the nullish values (undefined and null). Therefore, when we use this operator with undefined or null, it will return the fallback value (right-hand side value), as you can see in the image.
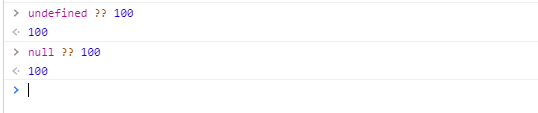
When we use this operator with other falsy values such as zero, false, NaN, etc., then it will return the left-hand side values, which you can see in the image.
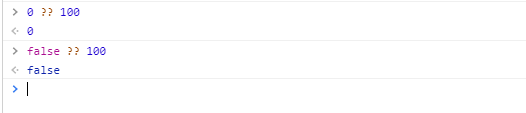
Example:
In the given example, there is an object name person
having two properties name
and age
. First, we have compared the value of these two properties with fallback values using logical OR operator. Then we have compared the same properties using nullish coalescing operator.
<!DOCTYPE html>
<html>
<head>
<title>Nullish Coalescing</title>
</head>
<body>
<script type="text/javascript">
let person = {
profile: {
name: "",
age: 0
}
};
console.log(person.profile.name || "Anonymous");
console.log(person.profile.age || 18);
console.log(person.profile.name ?? "Anonymous");
console.log(person.profile.age ?? 18);
</script>
</body>
</html>
Output:
As we can see in the output image the first two outputs are right-hand side values. This happens because the logical OR operator ||
returns the right operand if the left operand is falsy while the nullish coalescing operator ??
returns falsy values if the left operand is neither null nor undefined.
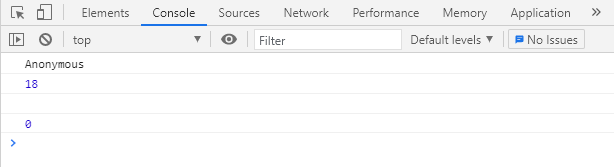
Conclusion
Nullish coalescing operator is the expansion of the logical OR operator. This operator only checks whether the left operand is null or undefined.