LAST UPDATED ON: SEPTEMBER 17, 2024
Pointer and Arrays in C
Before you start with Pointer and Arrays in C, learn about these topics in prior:
When an array in C language is declared, compiler allocates sufficient memory to contain all its elements. Its base address is also allocated by the compiler.
Declare an array arr
,
int arr[5] = { 1, 2, 3, 4, 5 };
Suppose the base address of arr
is 1000 and each integer requires two bytes, the five elements will be stored as follows:
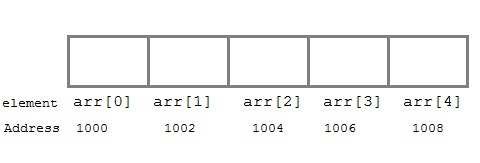
Variable arr
will give the base address, which is a constant pointer pointing to arr[0]
. Hence arr
contains the address of arr[0]
i.e 1000
.
arr
has two purpose -
- It is the name of the array
- It acts as a pointer pointing towards the first element in the array.
arr
is equal to &arr[0]
by default
For better understanding of the declaration and initialization of the pointer - click here. and refer to the program for its implementation.
NOTE:
- You cannot decrement a pointer once incremented.
p--
won't work.
Pointer to Array in C
Use a pointer to an array, and then use that pointer to access the array elements. For example,
#include<stdio.h>
void main()
{
int a[3] = {1, 2, 3};
int *p = a;
for (int i = 0; i < 3; i++)
{
printf("%d", *p);
p++;
}
return 0;
}
1 2 3
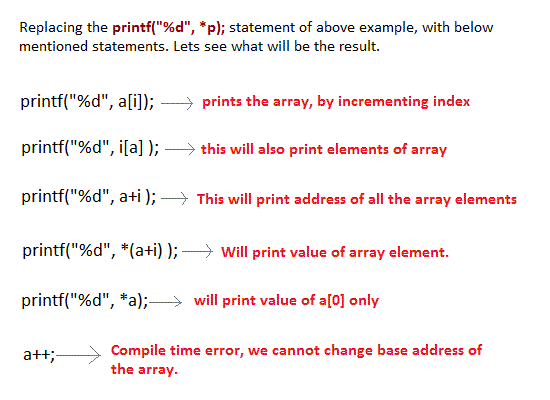
Here is the syntax:
*(a+i) //pointer with an array
The above code is same as:
a[i]
Pointer to Multidimensional Array in C
Let's see how to make a pointer point to a multidimensional array. In a[i][j]
, a
will give the base address of this array, even a + 0 + 0
will also give the base address, that is the address of a[0][0]
element.
Here is the syntax:
*(*(a + i) + j)
Pointer and Character strings in C
Pointer is used to create strings. Pointer variables of char
type are treated as string.
char *str = "Hello";
The above code creates a string and stores its address in the pointer variable str
. The pointer str
now points to the first character of the string "Hello".
- The string created using
char
pointer can be assigned a value at runtime.
char *str;
str = "hello";
- The content of the string can be printed using
printf()
and puts()
.
printf("%s", str);
puts(str);
str
is a pointer to the string and also name of the string. Therefore we do not need to use indirection operator *
.
Array of Pointers in C
Pointers are very helpful in handling character arrays with rows of varying lengths.
char *name[3] = {
"Adam",
"chris",
"Deniel"
};
//without pointer
char name[3][20] = {
"Adam",
"chris",
"Deniel"
};
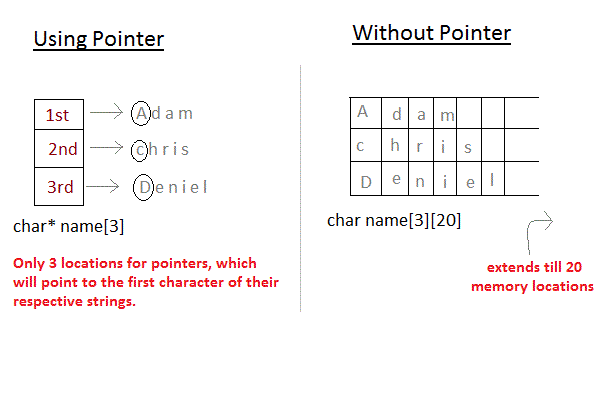
In the second approach memory wastage is more, hence it is preferred to use pointer in such cases.
Suggested Tutorials: